source code :
#include <iostream>
#include <GL/glut.h>
#include <math.h>
//LINGKARAN
const double PI = 3.141592653589793;
void drawCircle(float x_tengah,float y_tengah,float radius){
glBegin(GL_POLYGON);
int jumlah_titik = 50;
for (int i=0;i<=360;i++)
{
float sudut = i*(2*PI/jumlah_titik);
float x = x_tengah + radius*cos(sudut);
float y = y_tengah + radius*sin(sudut);
glVertex2f(x,y);
}
glEnd();
//sikil kanan tengah
glPushMatrix ();
glLineWidth (1.9f);
glBegin (GL_LINES);
glBegin (GL_POLYGON);
glColor3f (1.0, 1.0, 1.0);
glVertex3f (0.70, 0.00, 0.0);
glVertex3f (0.25, 0.00, 0.0);
glVertex3f (0.25, 0.00, 0.0);
glVertex3f (0.25, 0.02, 0.0);
glVertex3f (0.25, 0.02, 0.0);
glVertex3f (0.70, 0.02, 0.0);
glVertex3f (0.70, 0.02, 0.0);
glVertex3f (0.70, 0.00, 0.0);
glEnd ();
//sikil kanan atas
glPushMatrix ();
glLineWidth (1.9f);
glBegin (GL_LINES);
glBegin (GL_POLYGON);
glColor3f (1.0, 1.0, 1.0);
glVertex3f (0.60, 0.40, 0.0);
glVertex3f (0.05, 0.20, 0.0);
glVertex3f (0.05, 0.20, 0.0);
glVertex3f (0.05, 0.18, 0.0);
glVertex3f (0.05, 0.18, 0.0);
glVertex3f (0.60, 0.38, 0.0);
glVertex3f (0.60, 0.18, 0.0);
glVertex3f (0.60, 0.40, 0.0);
glEnd ();
//sikil kanan bawah
glPushMatrix ();
glLineWidth (1.9f);
glBegin (GL_LINES);
glBegin (GL_POLYGON);
glColor3f (1.0, 1.0, 1.0);
glVertex3f (0.60, -0.45, 0.0);
glVertex3f (0.05, -0.20, 0.0);
glVertex3f (0.05, -0.20, 0.0);
glVertex3f (0.05, -0.18, 0.0);
glVertex3f (0.05, -0.18, 0.0);
glVertex3f (0.60, -0.43, 0.0);
glVertex3f (0.60, -0.18, 0.0);
glVertex3f (0.60, -0.45, 0.0);
glEnd ();
//sikil kiri tengah
glPushMatrix ();
glLineWidth (1.9f);
glBegin (GL_LINES);
glBegin (GL_POLYGON);
glColor3f (1.0, 1.0, 1.0);
glVertex3f (-0.70, 0.00, 0.0);
glVertex3f (-0.25, 0.00, 0.0);
glVertex3f (-0.25, 0.00, 0.0);
glVertex3f (-0.25, 0.02, 0.0);
glVertex3f (-0.25, 0.02, 0.0);
glVertex3f (-0.70, 0.02, 0.0);
glVertex3f (-0.70, 0.02, 0.0);
glVertex3f (-0.70, 0.00, 0.0);
glEnd ();
//sikil kiri atas
glPushMatrix ();
glLineWidth (1.9f);
glBegin (GL_LINES);
glBegin (GL_POLYGON);
glColor3f (1.0, 1.0, 1.0);
glVertex3f (-0.60, 0.40, 0.0);
glVertex3f (-0.05, 0.20, 0.0);
glVertex3f (-0.05, 0.20, 0.0);
glVertex3f (-0.05, 0.18, 0.0);
glVertex3f (-0.05, 0.18, 0.0);
glVertex3f (-0.60, 0.38, 0.0);
glVertex3f (-0.60, 0.18, 0.0);
glVertex3f (-0.60, 0.40, 0.0);
glEnd ();
//sikil kiri bawah
glPushMatrix ();
glLineWidth (1.9f);
glBegin (GL_LINES);
glBegin (GL_POLYGON);
glColor3f (1.0, 1.0, 1.0);
glVertex3f (-0.60, -0.45, 0.0);
glVertex3f (-0.05, -0.20, 0.0);
glVertex3f (-0.05, -0.20, 0.0);
glVertex3f (-0.05, -0.18, 0.0);
glVertex3f (-0.05, -0.18, 0.0);
glVertex3f (-0.60, -0.43, 0.0);
glVertex3f (-0.60, -0.18, 0.0);
glVertex3f (-0.60, -0.45, 0.0);
glEnd ();
//jari kanan bawah
glPushMatrix ();
glLineWidth (1.9f);
glBegin (GL_LINES);
glBegin (GL_POLYGON);
glColor3f (1.0, 1.0, 1.0);
glVertex3f (0.60, -0.42, 0.0);
glVertex3f (0.58, -0.42, 0.0);
glVertex3f (0.58, -0.42, 0.0);
glVertex3f (0.58, -0.49, 0.0);
glVertex3f (0.58, -0.49, 0.0);
glVertex3f (0.60, -0.49, 0.0);
glVertex3f (0.60, -0.49, 0.0);
glVertex3f (0.60, -0.42, 0.0);
glEnd ();
//jari kiri bawah
glPushMatrix ();
glLineWidth (1.9f);
glBegin (GL_LINES);
glBegin (GL_POLYGON);
glColor3f (1.0, 1.0, 1.0);
glVertex3f (-0.60, -0.42, 0.0);
glVertex3f (-0.58, -0.42, 0.0);
glVertex3f (-0.58, -0.42, 0.0);
glVertex3f (-0.58, -0.49, 0.0);
glVertex3f (-0.58, -0.49, 0.0);
glVertex3f (-0.60, -0.49, 0.0);
glVertex3f (-0.60, -0.49, 0.0);
glVertex3f (-0.60, -0.42, 0.0);
glEnd ();
//jari kiri atas
glPushMatrix ();
glLineWidth (1.9f);
glBegin (GL_LINES);
glBegin (GL_POLYGON);
glColor3f (1.0, 1.0, 1.0);
glVertex3f (-0.60, 0.30, 0.0);
glVertex3f (-0.58, 0.30, 0.0);
glVertex3f (-0.58, 0.30, 0.0);
glVertex3f (-0.58, 0.39, 0.0);
glVertex3f (-0.58, 0.39, 0.0);
glVertex3f (-0.60, 0.39, 0.0);
glVertex3f (-0.60, 0.39, 0.0);
glVertex3f (-0.60, 0.30, 0.0);
glEnd ();
//jari kanan atas
glPushMatrix ();
glLineWidth (1.9f);
glBegin (GL_LINES);
glBegin (GL_POLYGON);
glColor3f (1.0, 1.0, 1.0);
glVertex3f (0.60, 0.30, 0.0);
glVertex3f (0.58, 0.30, 0.0);
glVertex3f (0.58, 0.30, 0.0);
glVertex3f (0.58, 0.39, 0.0);
glVertex3f (0.58, 0.39, 0.0);
glVertex3f (0.60, 0.39, 0.0);
glVertex3f (0.60, 0.39, 0.0);
glVertex3f (0.60, 0.30, 0.0);
glEnd ();
//jari kanan tengah
glPushMatrix ();
glLineWidth (1.9f);
glBegin (GL_LINES);
glBegin (GL_POLYGON);
glColor3f (1.0, 1.0, 1.0);
glVertex3f (0.70, 0.00, 0.0);
glVertex3f (0.68, 0.00, 0.0);
glVertex3f (0.68, 0.00, 0.0);
glVertex3f (0.68, -0.07, 0.0);
glVertex3f (0.68, -0.07, 0.0);
glVertex3f (0.70, -0.07, 0.0);
glVertex3f (0.70, -0.07, 0.0);
glVertex3f (0.70, 0.00, 0.0);
glEnd ();
//jari kiri tengah
glPushMatrix ();
glLineWidth (1.9f);
glBegin (GL_LINES);
glBegin (GL_POLYGON);
glColor3f (1.0, 1.0, 1.0);
glVertex3f (-0.70, -0.00, 0.0);
glVertex3f (-0.68, -0.00, 0.0);
glVertex3f (-0.68, -0.00, 0.0);
glVertex3f (-0.68, -0.07, 0.0);
glVertex3f (-0.68, -0.07, 0.0);
glVertex3f (-0.70, -0.07, 0.0);
glVertex3f (-0.70, -0.07, 0.0);
glVertex3f (-0.70, -0.00, 0.0);
glEnd ();
//mata kanan
glPushMatrix ();
glLineWidth (1.9f);
glBegin (GL_LINES);
glBegin (GL_POLYGON);
glColor3f (0.4, 0.4, 0.4);
glVertex3f (0.10, 0.43, 0.0);
glVertex3f (0.05, 0.43, 0.0);
glVertex3f (0.05, 0.43, 0.0);
glVertex3f (0.05, 0.45, 0.0);
glVertex3f (0.05, 0.45, 0.0);
glVertex3f (0.10, 0.45, 0.0);
glVertex3f (0.10, 0.45, 0.0);
glVertex3f (0.10, 0.43, 0.0);
glEnd ();
//mata kiri
glPushMatrix ();
glLineWidth (1.9f);
glBegin (GL_LINES);
glBegin (GL_POLYGON);
glColor3f (0.4, 0.4, 0.4);
glVertex3f (-0.10, 0.43, 0.0);
glVertex3f (-0.05, 0.43, 0.0);
glVertex3f (-0.05, 0.43, 0.0);
glVertex3f (-0.05, 0.45, 0.0);
glVertex3f (-0.05, 0.45, 0.0);
glVertex3f (-0.10, 0.45, 0.0);
glVertex3f (-0.10, 0.45, 0.0);
glVertex3f (-0.10, 0.43, 0.0);
glEnd ();
//mulut
glPushMatrix ();
glLineWidth (1.9f);
glBegin (GL_LINES);
glBegin (GL_POLYGON);
glColor3f (0.4, 0.4, 0.4);
glVertex3f (-0.02, 0.35, 0.0);
glVertex3f (0.02, 0.35, 0.0);
glVertex3f (0.02, 0.35, 0.0);
glVertex3f (0.02, 0.37, 0.0);
glVertex3f (0.02, 0.37, 0.0);
glVertex3f (-0.02, 0.37, 0.0);
glVertex3f (-0.02, 0.37, 0.0);
glVertex3f (-0.02, 0.35, 0.0);
glEnd ();
glFlush();
}
void display() {
drawCircle(0,0,0.3);
glColor3f (255/255, 255/255, 255/255);
drawCircle(0,0.40,0.15);
}
int main(int argc, char **argv){
glutInit(&argc,argv);
glutInitWindowSize(800,800);
glutInitWindowPosition(100,100);//jarak windows dari tepi monitor
glutCreateWindow("laba-laba");
glutDisplayFunc(display);
glutMainLoop();//mulai render
return 0;
}
Tuesday 11 November 2014
Gambar Laba-Laba Sederhana dengan OpenGL

Pakai kode disamping emoticon, terus tambahkan satu spasi sob..





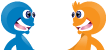





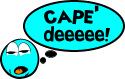




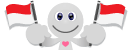




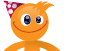






